in the post i will show you how to create your own ribbon button with the inventor api.
The code i use here can be found on my gibhub page
understand the UI concept
First of all we need to understand how the ribbons in inventor works. Inventor uses so called ‚Ribbons‘ to show you the nesseary UI. A full description of the concept can be found in the official documentation. According what you are doing you see different Ribbon. If you open a ‚Part‘ you see the ‚Part Ribbon‘, if you open a ‚Assembly Parts‘ you see the ‚Assembly Ribbon‘.
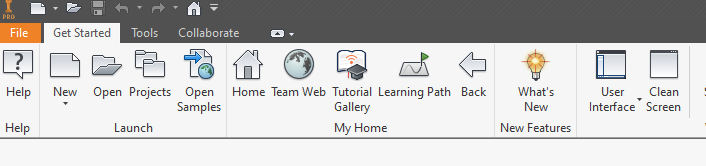
A full list of the ribbons and the attached tabs can be found here.
create a ribbon tab
in this example we are going to create a ribbon tab in the ’startup(ZeroDoc)‘ ribbon.
var ribbons = application.UserInterfaceManager.Ribbons; var ribbonName = "ZeroDoc"; var idMyOwnTab = "id_Tab_MyOwnTab"; RibbonTab ribbonTab; if (!RibbonTabExists(application.UserInterfaceManager, ribbonName, idMyOwnTab)) { ribbonTab = ribbons[ribbonName].RibbonTabs.Add("NoUseForAName", idMyOwnTab, addinId); } else { ribbonTab = ribbons[ribbonName].RibbonTabs[idMyOwnTab]; }
So if i run this we see –> nothing happens ! Maybe the application checks if the ribbon is empty. So now we add a tab to the ribbon!
create a ribbon panel
We need the id of the previous created ribbon tab.
if (RibbonPanelExists(application.UserInterfaceManager, ribbonName, idMyOwnTab, idMyOwnPanel)) return; var panel = ribbonTab.RibbonPanels.Add("MyPanel", idMyOwnPanel, addinId, "", false);
private static bool RibbonTabExists(UserInterfaceManager userInterfaceManager, string ribbonName, string tabName) { var ribbons = userInterfaceManager.Ribbons; foreach (RibbonTab tab in ribbons[ribbonName].RibbonTabs) { if (tab.InternalName == tabName) return true; } return false; }
add a command/button
After adding a panel and checking the output there is still no ribbon-tab and or a ribbon panel. So a command or button is missing. Creating a button is a whole chapter. Here is the needed code:
var buttonDescription = GetShowTextButton(addinId, application); panel.CommandControls.AddButton(buttonDescription);
The complete code should look like this:
private void CreateRibbonPanel(string addinId, Application application) { if (addinId is null) throw new ArgumentNullException(nameof(addinId)); if (application == null) throw new ArgumentNullException(nameof(application)); var ribbons = application.UserInterfaceManager.Ribbons; var ribbonName = "ZeroDoc"; var idMyOwnTab = "id_Tab_MyOwnTab"; var idMyOwnPanel = "id_Tab_MyOwnPanel"; RibbonTab ribbonTab; if (!RibbonTabExists(application.UserInterfaceManager, ribbonName, idMyOwnTab)) { ribbonTab = ribbons[ribbonName].RibbonTabs.Add("NoUseForAName", idMyOwnTab, addinId); } else { ribbonTab = ribbons[ribbonName].RibbonTabs[idMyOwnTab]; } if (RibbonPanelExists(application.UserInterfaceManager, ribbonName, idMyOwnTab, idMyOwnPanel)) return; var panel = ribbonTab.RibbonPanels.Add("MyPanel", idMyOwnPanel, addinId, "", false); var buttonDescription = GetShowTextButton(addinId, application); panel.CommandControls.AddButton(buttonDescription); }
Boom now it works 😀 The Result looks like this
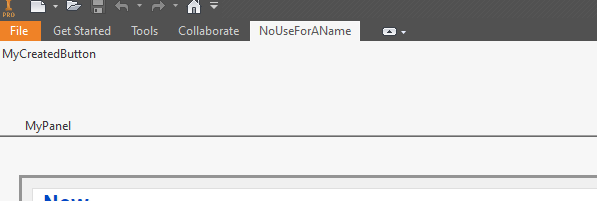
All the sourcecode can be found in my github reposetory. How to create a button aka the button-description can be found here