intension
in the previous post i showed how to create own ribbons, tabs, and buttons with the inventor api. In this post i will show you how you can define a button and automate the creation.
A lot of the code was taken from the Autodesk examples and from other websites. All my code can be found on github
create/define a button
the api needs a button description so that it can add something to a ribbon tab. Inventor has a command manager which holds all different commands.
- Clientid –> AddinId
- ButtonDisplayType
- NoTextWithIcon
- AlwaysDisplayText
- DisplayTextInLearningMode
- CommandTypes
- ShapeEditCmdType
- QueryOnlyCmdType
- FileOperationsCmdType
- FilePropertyEditCmdType
- UpdateWithReferencesCmdType
- NonShapeEditCmdType
- EditMaskCmdType
- ReferencesChangeCmdType
- SchemaChangeCmdType
The following code sample was modified from the ‚SimpleAddin‘ Autodesk Inventor example.
buttonDefinition = app.CommandManager.ControlDefinitions.AddButtonDefinition( ibdc.DisplayName, ibdc.InternalName, ibdc.CommandType, ibdc.ClientId, ibdc.Description, ibdc.Tooltip, PictureDispConverter.ToIPictureDisp(ibdc.StandardIcon), PictureDispConverter.ToIPictureDisp(ibdc.LargeIcon), ibdc.ButtonDisplayType); buttonDefinition.Enabled = true; buttonDefinition.OnExecute += ButtonDefinition_OnExecute;
I modified the example so that it fits to my needs. I wanted a reusable, clean and easy to use button description.
How to create/define a button :
public class TestButton : Button { private readonly string addinId; public TestButton([NotNull] Application application, string addinId) : base(application) { this.addinId = addinId; } protected override ButtonDescriptionContainer GetButtonDescription() { return new ButtonDescriptionContainer() { ButtonDisplayType = ButtonDisplayEnum.kNoTextWithIcon, CommandType = CommandTypesEnum.kShapeEditCmdType, Description = "Description", InternalName = "InternalName", DisplayName = "MyCreatedButton", ClientId = addinId, Tooltip = "this should be a ToolTip" }; } protected override void ButtonDefinition_OnExecute(NameValueMap context) { var frm = new ShowTextFrm(); frm.ShowDialog(); } }
usage :
. .. var buttonDescription = GetShowTextButton(addinId, application); panel.CommandControls.AddButton(buttonDescription); .................. private ButtonDefinition GetShowTextButton(string addinId, Application application) { CommandCategory slotCmdCategory = application.CommandManager.CommandCategories.Add("Slot", "Autodesk:YourAddIn:ShowTextCmd", addinId); var btn = new TestButton(application, addinId); slotCmdCategory.Add(btn.ButtonDefinition); return btn.ButtonDefinition; }
/// <summary> /// taken from the inventor 'SimpleAddIn' Sample /// </summary> public abstract class Button { private ButtonDefinition buttonDefinition; protected abstract ButtonDescriptionContainer GetButtonDescription(); public ButtonDefinition ButtonDefinition => buttonDefinition; protected Button([NotNull] Application application) { Initialize(application); } private void Initialize([NotNull] Application app) { if (app == null) throw new ArgumentNullException(nameof(app)); var descriptionContainer = GetButtonDescription(); if (descriptionContainer is IconButtonDescriptorContainer ibdc && ibdc.StandardIcon != null && ibdc.LargeIcon != null) { buttonDefinition = app.CommandManager.ControlDefinitions.AddButtonDefinition( ibdc.DisplayName, ibdc.InternalName, ibdc.CommandType, ibdc.ClientId, ibdc.Description, ibdc.Tooltip, PictureDispConverter.ToIPictureDisp(ibdc.StandardIcon), PictureDispConverter.ToIPictureDisp(ibdc.LargeIcon), ibdc.ButtonDisplayType); } else { buttonDefinition = app.CommandManager.ControlDefinitions.AddButtonDefinition( descriptionContainer.DisplayName, descriptionContainer.InternalName, descriptionContainer.CommandType, descriptionContainer.ClientId, descriptionContainer.Description, descriptionContainer.Tooltip, Type.Missing, Type.Missing, descriptionContainer.ButtonDisplayType); } buttonDefinition.Enabled = true; buttonDefinition.OnExecute += ButtonDefinition_OnExecute; } protected abstract void ButtonDefinition_OnExecute(NameValueMap context); }
If you run this example your button should look like this
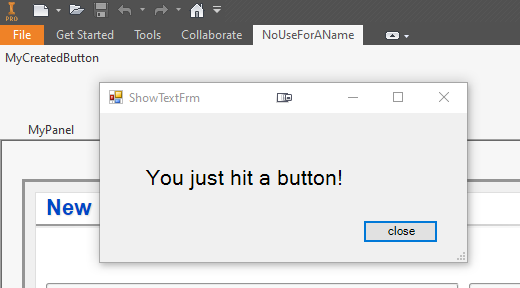
Pingback: create a ribbon buttons/commands with the inventor api - coding for fun
Hi, my name is Hoang, i am so impressed with all the thing that you have done with the inventor api. I followed the your instruction and got something done. I got new buttons my self, but just on ribbon tab and pannel that already exist, and of course OnExcute of that button work normally. Until i apply your instruction, i can create my own ribbon tab and pannel, then i put my new button on it. The problem is when i have that new ribbon, every button that i add by the way i did before somehow do not work, OnExcute of these button can not be called each time i click on it. Only when i add your Button class and your Test Button class and use them, it work. Hope your can show me what problem that i am facing, why can not i overload OnExcute normally. Wait for your respond Thank you so much
Hallo Hoang,
thanks for reaching out. Based on your description i cannot find anything wrong. It would be nice if you can provide any sample code (github ? or info@codingforfun.de).
What i can assume ist, that the name and the id of each panel/button needs to be unique !
buttonDefinition must be class variable, worked for me with same issue
[GuidAttribute(„69B0E76B-C139-41C7-A88F-030ED1E19448“), ComVisible(true)]
public class AddIn : ApplicationAddInServer
{
// Inventor application object.
private Application _mInventorApplication;
// Не знаю почему – но Definition это Class Variable
private ButtonDefinition _buttonDefinition; // like this